Learn CSS Variables - CSS Custom Properties to Make Your CSS Reusable
In this post you will learn what are CSS variables and how you can use them.
So what are CSS variables you can understand from the name. You can understand variables inside CSS like inside programming language. And essentially CSS is not a programming language. This is why it is extremely important that we use there at least variables so our styles inside are reusable and supportable.
Can is use it everywhere?
And the first question is if we can safely use CSS variables everywhere and what browsers are supported.
Here I'm inside Can I use
and you can see CSS Variables here which are custom properties inside CSS. As you can see we can safely use them if you don't need to support old browsers like IE11 for example. Typically all companies nowadays support at least Edge browser so you are safe here.
How to use them?
Let's say that we have such markup.
<div class="container">
<button>Hello Vars</button>
</div>
Now let's write styles for our button.
.button {
color: var(--button-color, gray);
}
Here we wrote CSS for .button
and provided color inside. But instead of value we used var(--name)
construction. This is exactly how we use variables in CSS. So it is not creation but usage. The second argument is the default value so if color is not provided for our button it will have gray color.
Now the question is how we can provide a variable to our button. We can do that by defining it in parent container.
.container {
--button-color: red;
}
.button {
color: var(--button-color, gray);
}
As you can see our colors on the button are red now and you can directly see our variable in inspector.
Just a hint for you. If you can't hover on the variable in inspector then it is not provided.
But it is important to remember that the definition of the variable with a color inside .container
doesn't do anything at all. It simply defines a variable but it doesn't apply it anywhere. Which actually means that you always want to use a combination of defining the variable and using it.
Scopes
Now it's time to talk about scopes. Previously we define our color inside a container.
.container {
--button-color: red;
}
Which means this variable is isolated inside the container and is available to all it's children.
Typically in big applications people like to create all their variables in a single place and use it across the whole application. And in order to do that we can use :root
.
:root {
--button-color: red;
}
As you can see in browser it is still working like before but now the variable comes from root.
Root means that everything inside our document will have access to this variable.
You can imagine just a single file with hundreds or thousands different CSS variables and we are reusing them everywhere. So example you can define such things.
:root {
--button-color: red;
--padding-sm: 10px;
--padding-md: 20px;
}
Then across your application instead of just number you can use variables. It helps tremendously because you just need to update one variable and the whole project is changed.
Additionally you must remember that it doesn't matter how much you will nest your markup. The variable will be available for all it's children.
.container {
--button-color: red;
}
.some-super-deep-nested-class {
color: var(--button-color, gray);
}
Where can I use it?
One more thing to remember is that you can use them in the default values as well.
.container {
--button-color: red;
}
.button {
color: var(--button-color, var(--button-color-default, gray));
}
In this case if --button-color
is not provided then --button-color-default
is used. If both are not provided then we fallback to the default.
But it makes it unreadable so I won't recommend you to use such construction.
CSS Variables in frameworks
The last thing to talk about is frameworks. Inside all popular frameworks like React, Angular or Vue all your styles are isolated. Now you for sure have a question if it makes sense to use CSS Variables in frameworks.
This is a tricky question as this is something that you are not typically using as a recommendation from the frameworks. Frameworks give you possibilities to configure your components. We are not talking just about styling in these frameworks but and components.
Which actually means that we are using props inside React or inputs inside Angular.
This is much better to stick to the frameworks than to use CSS variables there. Because in that case it will be kind of hack. These CSS variables functions outside of your frameworks.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done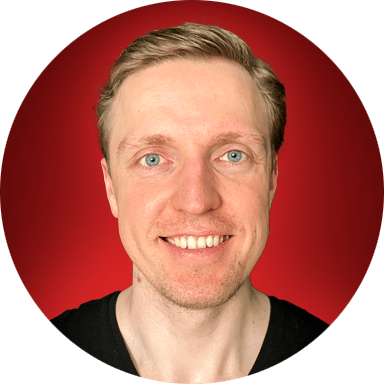