Learn Temporal API In 10 Minutes
In this post I want to show you a new way to work with dates inside Javascript.
And actually this is not fully true. The thing that I want to talk about is called Temporal and it is not released yet. It is in the third stage of proposal to be added to Javascript.
Here we have a new way to work with Javascript and it is called Temporal. This proposal is currently in Stage 3 but I think that we will get it in production really soon because this is a copy paste of most popular libraries to work with dates and this is nothing new or untested.
What do we have?
Working with dates in plain Javascript is a huge pain. This is why almost all people are using 2 most popular libraries: Luxon and Date-fns.
Here is an example of Luxon library.
DateTime.now().setZone('Europe/Berlin').minus({weeks: 1}).endOf('day').toISO()
Even if you never used Luxon you can guess what happens here. We get dates now, set a timezone, subtract 1 week, get end of day and convert to ISO string.
This is extremely powerful and completely immutable. This is why I highly recommend you this library if you are working with dates. And this is my preferred choice.
If you don't like Luxon we have similar alternative which is called Date-Fns. It is another popular library where we get nice API to work with dates inside Javascript. It also supports timezones out of the box.
Do we have a problem?
What's the problem then? The problem is that this is the core functionality of Javascript and all people need working with dates. And we don't have it in Javascript out of the box. Because native implementation of working with dates is really bad.
This is why the core team of Javascript decided to work on Temporal API for native support inside Javascript. And I'm almost sure that it will be approved. Why? If you will look on Luxon library and on Temporal API you will see that it's 99% the same.
Which actually means that we didn't reinvent the wheel, we just took a super popular library and we wrote exactly the same. But you must understand that this functionality is not yet available in any browser. If you want to use it in your project you can either install a polyfill (@js-temporal/polyfill)
npm install @js-temporal/polyfill
and then use as
import {Temporal} from '@js-temporal/polyfill'
But if you just want to check it out you can just to official page and test it here. In this website Temporal is available inside window object.
And actually there is a lot of stuff to work with in Temporal. We have here working with dates, converting from ISO, to ISO, generating date objects, working with timezones.
This is why here I just want to show you several examples that I use every single day when I work with dates.
Working with current date
First let's look how we can get a current date. This functionality we need for sure. If we write Temporal inside console we will see lot's of different namespaces like Calendar, Duration, TimeZone.
And this is exactly what we have for example in Luxon. For example if we want to work with durations we will for sure take Temporal.Duration namespace.
So how we can get current date?
// Date now
Temporal.Now.instant()
As a result we get a special Instant which is an object of Temporal. This is now plain Javascript string. It is an instance of our Instant class.
But actually this format is not suitable for us to work in our applications. This is why we want to call toString()
on it.
// Date now
Temporal.Now.instant().toString()
This gives us a nice UTC string which we typically get from backend.
Working with ISO string
The next case that you will for sure have is converting our UTC string to Temporal object. Why do we need that? Typically we store inside backend all our dates like UTC strings. This is why we want to convert it Temporal date to apply different methods to it.
For this we can write
// from ISO to Temporal
Temporal.Instant.from('1969-07-20T20:17Z')
As you can see we are getting again our date with Instant class of Temporal.
Date with timezone
Another case will be to generate a date with timezone of the user this is set in browser. For this we can use Temporal.ZonedDateTime
and provide a timezone of the user inside.
// generate date with timezone
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).toString()
Here we used method ZonedDateTime.from
were we can provide an object with different fields and timezone. It will generate a valid Temporal date with timezone of the user.
And again there is nothing new here. For example inside Luxon we have a method from which does exactly the same.
Luxon.DateTime.from({year: 2022, day: 1, month: 1}, {zone: 'Europe/Berlin'})
Reading information
For example let's say that we need to get day of week in some Temporal date.
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).dayOfWeek
We simply write dayOfWeek
and get this information. The same is to get days in month.
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).daysInMonth
or days in year.
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).daysInYear
Add & Subtract
Another important usecase for me is to add and subtract information from dates.
Here is how we can add some period to our Temporal date.
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).add({days: 3})
We can provide any time range like days, months, minutes or years.
And here is subtract of days.
Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"}).subtract({days: 3})
Difference
And the last feature that I want to show you is the difference. Sometimes we must calculate a range between 2 dates in specific format. For example in days, months or years.
const date2 = Temporal.ZonedDateTime.from({year: 2022, day: 1, month: 1, timeZone: "Europe/Berlin"})
const date1 = date2.subtract({days: 3})
date2.since(date1, {largestUnit: 'year'}).days
Here we got an interval between 2 dates in days.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
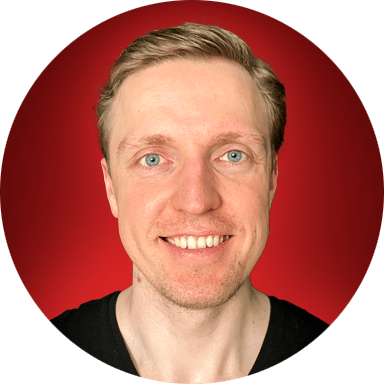