Local Storage, Session Storage or Cookies? XSS and CSRF included.
In this post I want to compare three different places where we can store data in the frontend and it is session storage, local storage and cookie.
And the most common question that I'm getting from beginners is something like this: "Ok I'm developing React application or maybe Javascript application and when I'm reloading the page all my data are erased and everything is empty. What can I do about it?". Actually you must remember that inside Javascript we store all our variables and all our logic just inside memory which actually means every single time when you reload the page everything is erased and you start from the beginning. This is how Javascript works and you can’t really change it. Period.
So typical applications are working like this - after every page reload everything is erased, we fetch all needed data from the API and generate our website again. Which actually means all our data is removed in any case.
But we also have another approach. If you don’t want to bother with API, backend or databases than you can store data locally inside browser. It is completely possible but it is not a production solution because for production you typically want to store most of your data inside the database. It is not enough to just store your data inside browser because they can be erased and can't be validated. So, these solutions are just for local development purposes, just to test something really quickly or just to store some additional properties on the client side additionally to your database in production. So, we have here three possibilities: we have local storage, session storage and cookies.
Local storage
Let’s start with the local storage. Actually, inside window
as you can see here we have a property which is called localStorage
. And as you can see this is a class storage.
What we want to do here, we typically want two things. First we want to write something to local storage and secondly read something from it. For this we use localStorage.setItem
and it will set a key inside local storage.
Also you must remember that everything inside local storage is stored as a string and as a key and value.
Which actually means that here as you can see we must provide key and value.
localStorage.setItem('foo', 'bar')
For example here we set key as foo and our value as bar. What does it mean? This line will set inside local storage our new key value pair. And actually now inside Chrome Devtools we can check that we successfully created our new key inside local storage. And for this you must open application tab and after this on the left we have storage section and local storage.
As you can see now we have our new key with foo and value bar. And, actually here we can see all our keys which are related to this web site.
The next question is how we can read our pair from local storage. And for this we are using localStorage.getItem
and here we must provide our unique key, which is foo
.
localStorage.getItem('foo')
And as you can see we are getting back our string bar. And it is important to remember that we can store there only strings, which actually means if you want to store for example arrays or objects which you typically want then we must stringify this data first.
localStorage.setItem('foo', JSON.stringify([1, 2, 3]))
Here we save an array to local storage, but we are not providing array inside, we must use JSON.stringify
first to transform our array in the string. As you can see now inside our application here, we have a string one two three.
When we use here localStorage.getItem
you can see it is not an array. It is stringified array, which is a string. Which actually means in this case if you want to get your data back, you must parse them with JSON parse
.
JSON.parse(localStorage.getItem('foo'))
So, here we can write JSON.parse
and we are getting our array back which is exactly what we wanted. So, these are two most popular commands that you will use with local storage.
You must use local storage if you simply want to store some property persistent between page reloads and you don’t want to store this information inside your backend, API or server.
Now, let’s talk about some PROS and CONS.
- First of all local storage is supported in almost all browsers (at least modern browsers) which means we are on the safe side here.
- But here also some limitations of local storage. First of all it can’t be bigger than 5 MB per domain. And actually 5MB is quite a lot but it may be not enough in some case.
- Secondly, it is not supported in all browsers for example in IE8 and earlier but typically it doesn't matter for you because you are supporting only modern browsers.
- It is not related to your server in any way. You are storing just this data inside local storage and they are available only on frontend. You can’t really get access to this data in any way inside your HTTP request and this is important to remember.
So, your local storage data is completely isolated inside client, which actually means we store there just some frontend data which are not sensitive information. Why not sensitive? Because actually this data is available inside Javascript. And it means that it is available for XSS attack. If you don’t know what is XSS, this is when somebody is calling some Javascript code inside your browser for example. And actually in this case they can read all your local storage, for example your authentication token or do some request to the backend with your information. This is why it is super important that you avoid storing in any personal information, security information or tokens inside local storage.
Session storage
The next thing to talk about is session storage. And actually this is something that you can simply forget because session storage is exactly a copy paste of local storage, but it exists only inside current tab until you close this tab. Which actually means typically nobody is using it at all. And actually here you can see that we have
sessionStorage.setItem('foo', 'bar')
sessionStorage.getItem('foo')
For example here we used setItem
and we stored bar
inside foo
. It is working exactly the same as localStorage. Now we can jump here inside our application and check on the left not local storage, but session storage.
And as you can see here we have this key, but it doesn’t make a lot of sense to use this feature, because it is not persistent when you close and open your browser. And this is what you typically want. You want to store some information even when user closes the browser.
Which means local storage and session storage are identical, but we almost always are using local storage.
Cookies
And the last thing which is the most interesting is cookie. So, what is cookie? This is also some kind of storage, but it is not directly a place, where you store something. So, actually you can set a cookie on the server and on the client and then this cookie will be transported with your every single request from your client to your server.
As you can see here I’m logged in inside twitter and inside my application cookies, I have lots of cookies, which actually means typically all these cookies were generated on the backend and this means that all these cookies are attached to every single request that were making inside this system from the client to the server.
This is why here I can jump inside network, select some request and you can see here I have the request api.twitter update subscriptions and here on the tap cookies you can see all cookies, which are attached to this specific request.
So, this is actually the main difference, it is not really a storage like for example local storage, but a way to store some information securely inside browser, which is available directly on the client and on the server.
And the idea is that actually you can set cookie on the server and on the client, but typically we want to save our cookie secure and we don't want to allow access in this cookies and modify in them inside client. And for this we have two properties. We can set a cookie with key HttpOnly
and with key secure
. Secure means that this cookie will be only transferred through HTTPS, which is extremely save and HTTPOnly means that this cookie will be available only for server and not for client. And this is extremely secure.
Which actually means if you have some tokens or sensitive information, you always want to use for these cookies and not local storage and secondly you want to use these two keys HTTPOnly and Secure so you make it really safe for your application.
As you can see all these cookies are attached with the request and here we see our table with HTTPOnly and Secure. And you can see that checkboxes are checked in some cookies.
Now here I also must tell you about CSRF attacks. What does it mean? It means that you are opening some email for example from somebody, and you simply click on that link. The main problem is how the web is working at all. Actually if the origin is the same inside this URL, then all cookies will be attached to your request.
This is how web is working if the origin is the same, than all cookies are attached directly.
Which actually means if you are logged in on some web site and you simply click on some link, then something bad can happen to you, because you are logged in with your account, which actually means you are making something with your specific account, by your authentication inside cookie.
So, I highly recommend you not to open some strange emails and links from people that you don’t know.
So, what a pros and cons of cookies?
- Actually is not really a storage, this is a place to store some data to communicate between client and server.
- Also you must understand that all these cookies is simply just a single string. Which actually means we can’t really store a lot of information there.
- But also it is really good that inside cookies we have this HTTPOnly and Secure flags, which makes cookies really amazing for tokens and sensitive information.
- We also have expired date on the cookies which means we can set expiration date inside cookie and it helps also a lot.
- And as I already said cookies are limited in size, it is just 4 Kb maximum, which actually means it is not exactly the place, where you can store lots of data.
Conclusion
So here is a conclusion you really don’t need to learn session storage and you don’t need to use it. Secondly, you will use local storage typically if you want to store some data between page reloads. And you only want to do it client side without any API or backend at all. And actually if you want something secure for your sensitive information or you want to make this information available for the client and the server with every single request than cookie is your best bet.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
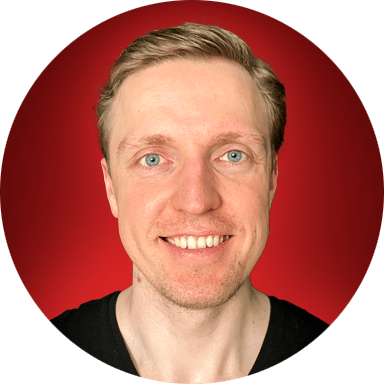