Navbar HTML Css Javascript | Sidebar Menu 4 Variants
In this post I want to show you 4 different variants how you can implement toggling of your sidebar with just plain CSS, HTML and Javascript.
So this is what I prepared for us here.
We have our sidebar without any styles: this is just a close button and 4 links. We also have our main section and toggle sidebar button.
This is how our html looks like.
<div class="sidebar">
<span class="closeSidebarButton">×</span>
<a href="#">Home</a>
<a href="#">Courses</a>
<a href="#">Posts</a>
<a href="#">Contact</a>
</div>
<div class="main">
<h2>Test sidebar</h2>
<p>Click on the element below to show side navigation menu</p>
<span class="toggler">☰ open</span>
</div>
Styling sidebar
Now let's add some styling to our sidebar.
.sidebar {
height: 100%;
width: 0;
position: fixed;
z-index: 1;
top: 0;
left: 0;
background-color: #111;
padding-top: 60px;
transition: 0.5s;
overflow-x: hidden;
}
It's a fixed container which must go on the top of the content. Most importantly we set width
to zero because by default our sidebar must be hidden.
Now let's style links inside our sidebar.
.sidebar a {
padding: 8px 8px 8px 32px;
text-decoration: none;
font-size: 25px;
color: #818181;
display: block;
transition: 0.3s;
}
.sidebar a:hover {
color: #f1f1f1;
}
This is how our sidebar looks now.
But if you want to see it you must change "just for testing" width
to 250px
.
Toggling sidebar
Now is the question how to will toggle our sidebar with Javascript. And actually we can do it in different way. First of all we could directly change width style on our element with Javascript. But it is not the best approach.
What I really like is to put all my states inside CSS. So all our states are predefined and we just add or remove a class.
This is why in our css I want to create styles for opened sidebar.
.sidebar.is-opened {
width: 250px;
}
Now we can add a event listener for our sidebar inside Javascript.
const $toggler = document.querySelector(".toggler");
const $sidebar = document.querySelector(".sidebar");
const $main = document.querySelector(".main");
const $closeSidebarButton = document.querySelector(".closeSidebarButton");
$toggler.addEventListener("click", () => {
$sidebar.classList.toggle("is-opened");
});
Here before we added event listener I first saved all our elements to variables in order to use them later. We all used classList.toggle
to implement adding or removing of the class on the sidebar.
As you can see in browser now we can open as close our sidebar.
But we also have a cross in our sidebar so we must handle it also.
$closeSidebarButton.addEventListener("click", () => {
$sidebar.classList.remove("is-opened");
});
Move content
We implemented the first variant of toggling but sometimes you want to move your content so that sidebar never overlap our content.
This is why I want to style our main containter when we have is-opened
class.
.sidebar.is-opened + .main {
margin-left: 250px;
}
This will change the sibling of is-opened
which is .main
. So when we apply is-opened
class we directly change our .main
.
As you can see it is moved now.
But it would be nice to add transition to both sidebar and main to look smooth. We already have transition on our sidebar but not on main
.sidebar.is-opened + .main {
margin-left: 250px;
transition: margin-left 0.5s;
}
Blurring content
Another variant that you might want to implement is blurring content after opening a sidebar. And we can also do it with CSS.
.sidebar.is-opened + .main {
margin-left: 250px;
transition: margin-left 0.5s;
background: rgba(0, 0, 0, 0.4);
}
.main {
height: 100vh;
padding: 25px;
}
So we added here background with opacity to .main
when our sidebar is opened. We also set some default style for main to look better.
Hiding content
You might also want to hide content completely and just show sidebar. For this we just need to set sidebar to full width and center our links.
.sidebar.is-opened {
width: 100%;
text-align: center;
}
This is how it looks like.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done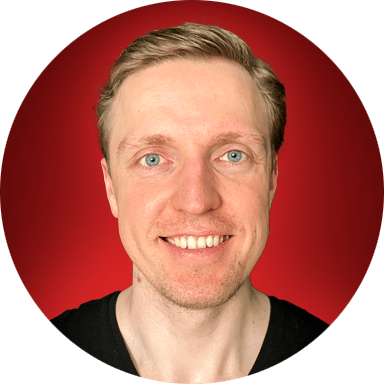