Sort Array Method - You Need to Know This Cases
In this post we will talk about sorting is Javascript. This is considered a basic knowledge but there are still quite a lot of things that are not that easy inside sorting.
Mutating data
Inside Javascript we have just a single method sort and we can call it on the array.
array = ["Table", "Chair", "Glass", "Mouse", "Laptop", "Fan", "Car"];
array.sort()
console.log(array);
Here I have an array and we can call .sort()
on it which will sort our data in alphabetical order.
['Car', 'Chair', 'Fan', 'Glass', 'Laptop', 'Mouse', 'Table']
But here is an important point. We first of all called sort and after this logged our array. Which actually means that we didn't assign the result of the function anywhere.
Because actually sort function mutates the existing array
We don't need to assign the result anywhere. But actually this is super misleading.
console.log(array.sort());
Because here inside console.log
we can write array.sort()
and get a sorted array. The problem is that it gives a sorted result back but also mutates the existing array. This is why why you must always be aware that sort function will change your array.
How sorting works?
Another important point is to understand how sorting works exactly. Sorting function compares each element in our array one by one. But actually when it compares strings, then it compares them by first letter.
array = ["Table", "Chair", "Glass", "Mouse", "Laptop", "Fan", "Car"];
Here sorting function looks on first letter of word "Table" and first letter of word "Chair". If they are equal then it compares second letters. This is why it works with strings like a charm.
But it will never work correctly with string numbers.
array = ["200", "65", "5", "2"]
console.log(array.sort())
After sorting we get array like this
['2', '200', '5', '65']
Now you can understand why it happens. Because actually it compares strings symbol by symbol.
This is why you can't use sorting to sort array of numbers as strings
Sorting in descending order
Another question that people typically ask is "How we can sort in descending order?". By default sort function sorts in ascending order. There is no way to provide it inside. This is what people typically write.
array = ["Table", "Chair", "Glass", "Mouse", "Laptop", "Fan", "Car"];
array.sort()
console.log(array.reverse());
So after sorting the array they simply call reverse to reposition all elements in the array. But it is really bad because sort
is mutable function and reverse
is immutable. So it needs to be assigned to the new property. It won't change the old array. This is why I recommend you to never use such approach. It is better to use predicates for this.
Sorting with predicate
We can pass a function inside a sort
function. And there we must provide a predicate how we can compare our pairs.
numberArray = [2, 3, 4, 6, 66, 100, 25, 33];
numberArray.sort((a, b) => a - b);
console.log(numberArray);
As you can see in this case it is sorted in ascending order. a
and b
here are pairs of elements which we compare one by one. Our predicate must return a number less than zero and bigger than zero depending on how we want to sort it. This is why when we sort numbers we can write a - b
. If we need descending order we can write b - a
.
Sorting array of objects
Let's look like we can sort array of objects by predicate.
const people = [
{name:"Albert", yob:1997},
{name:"Dave", yob:2005},
{name:"John", yob:2000}
];
people.sort((a, b) => a.yob - b.yob);
Here we wanted to sort people by year of birth. This is exactly the same thing like we did with array of numbers.
But want to do if we want to sort strings?
const people = [
{name:"Albert", yob:1997},
{name:"Dave", yob:2005},
{name:"John", yob:2000}
];
people.sort((a, b) => a.name > b.name ? -1 : 1);
Here we compared name and returned -1 and 1 depending if it is true or false. As I said the result of our sort function should be negative number or positive number. Typically we return -1 and 1 when we check some logic inside.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
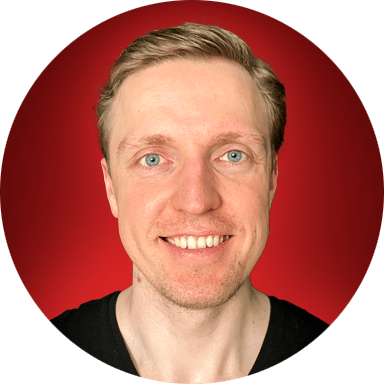