Ngx Bootstrap - Best Angular Components Library
In this post I want to show you a specific library for Angular which is called Ngx-Bootstrap and this is an amazing library with lots of predefined components that are difficult to implement on your own.
And actually I'm not a huge fan of using libraries because at some point you must update them, sometimes they don't do what is intended and they have lots of code inside that you don't need.
Additionally it is easier to support your own code than the library. But actually inside Angular I used Ngx-Bootstrap a lot.
Here is an official website which is not related to Angular project of Bootstrap team.
Here inside documentation we can see the list of components that we have. All this components are for Angular and this library heavily relies on Bootstrap as a dependency.
Actually almost all these components you will need in every single project. Most popular components here that I used a lot are: modals, pagination, dropdowns, date pickers and tooltips.
Why does it make sense to use a library and not to build all these components on our own? If we are talking about tooltip or modal (because that are super similar) this is a component that you want to render inside body.
Why inside body? If you just render it inside your component then you will have problems with CSS overflow because it can be cutted by other components. If we render it inside body we don't have such problems.
Inside Angular it is not that trivial to implement rendering of the component inside body. By using a library we get correct behaviour out of the box inside all these components.
Also all these components are well tested and they are really stable. So let's try to implement in our project several components and have an understanding how it all works together.
Installation
Here I already prepared for us an empty Angular application. Now let's install this package.
yarn add ngx-bootstrap
Here you must check if you get any errors. If you have older version of Angular you might get an error that the library is not compatible.
You must remember that Ngx-Bootstrap is tied to latest versions of Angular. Which actually means that at the moment of this video I must use Angular 14. In this case ngx-bootstrap won't throw any error.
After this we must import CSS from the Bootstrap library inside our project. The easiest way to do it is just to put it in index.html.
// src/index.html
<head>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css"
rel="stylesheet"
crossorigin="anonymous"
integrity="sha384-KyZXEAg3QhqLMpG8r+8fhAXLRk2vvoC2f3B09zVXn8CA5QIVfZOJ3BCsw2P0p/We"
/>
</head>
Tooltip module
Now we must import some module from ngx-bootstrap.
import { TooltipModule } from 'ngx-bootstrap/tooltip';
...
@NgModule({
imports: [
...
TooltipModule.forRoot(),
],
})
export class AppModule {}
As you can see we import a single module for specific component which allows us to still have a small bundle of usable features. Also Ngx-bootstrap is written in a way that we use forRoot
to provide configuration to the specific module.
We successfully injected our module, now we can use it in our app.component.html
.
<button
type="button"
class="btn btn-primary"
tooltip="Fooooo"
>
Checking tooltip
</button>
As you can see to use a tooltip on the button it is enough to add a tooltip
directive and it works.
And here are some important configurations for the tooltip.
Really often you want to show tooltip on click and not on hover. For the we can add a trigger.
<button
type="button"
class="btn btn-primary"
tooltip="Fooooo"
triggers="click"
>
Checking tooltip
</button>
Now our tooltip if being shows on click.
Another important thing that we want is appending of the tooltip to the body.
<button
type="button"
class="btn btn-primary"
tooltip="Fooooo"
container="body"
>
Checking tooltip
</button>
By setting container
to body
we won't have any problems with overflow in CSS.
And last thing about tooltips is obviously providing templates inside.
<button
type="button"
class="btn btn-primary"
[tooltip]="tooltip"
>
Checking tooltip
</button>
<ng-template #tooltip>Hello {{ username }}</ng-template>
Here instead of the string we provide inside ng-template
. It allows us to write custom markup with any variables from our class.
As you can see this library simplifies a lot implementation of tooltips.
Modals
Now let's look on modals. Modals is something that you need in every single project. First of all let's register our module.
import { ModalModule } from 'ngx-bootstrap/modal';
...
@NgModule({
imports: [
...
ModalModule.forRoot(),
],
})
export class AppModule {}
Now we can use it inside our component like we did with the tooltip.
<button type="button" class="btn btn-primary" (click)="openModal(modal)">
Open modal
</button>
<ng-template #modal>
<div class="modal-header">
<h4 class="modal-title pull-left">Modal</h4>
<button
type="button"
class="btn-close close pull-right"
aria-label="Close"
(click)="modalRef?.hide()"
>
<span aria-hidden="true" class="visually-hidden">×</span>
</button>
</div>
<div class="modal-body">This is a modal.</div>
</ng-template>
As you can see on the top we defined a button with click event which calls openModal
function and passes modal
inside.
After this we defined an ng-template
which is exactly a modal which we pass in the function. Inside we have a standard Bootstrap modal markup. The only thing that we have from ngx-bootstrap
here is modalRef?.hide()
. This is a possibility to manipulate the modal from the component.
This is why now we need to define openModal
logic in our component.
export class AppComponent {
modalRef?: BsModalRef;
constructor(private modalService: BsModalService) {}
openModal(template: TemplateRef<any>) {
this.modalRef = this.modalService.show(template);
}
}
Here we injected BsModalService
to be able to open a modal. Inside openModal
method we called show
where we passed the modal that we created in the template.
The most important part is that we got back this.modalRef
which is a reference to the open modal. This is exactly what allows us to call in the template modalRef?.hide()
.
As you can see in browser we can open a modal when we click on the button and correctly styled markup. Also close button inside our modal works correctly.
Also modal is being rendered by default inside body. This is why we are sure that we won't have any collisions with overflow:hidden.
Datepicker
The last component that I want to show you is date picker and date range picker. These are really difficult components that are not that easy to implement on your own. This is why it is so nice to get them from the library.
In order to use it we must add specific styles from ngx-bootstrap to style it correctly in index.html
<link
rel="stylesheet"
href="https://unpkg.com/ngx-bootstrap/datepicker/bs-datepicker.css"
/>
These are just styles directly for datepicker. If you don't plan to use this component you should not include it.
Our next step is to register module inside app.module.ts
.
import { BsDatepickerModule } from 'ngx-bootstrap/datepicker';
...
@NgModule({
imports: [
...
BsDatepickerModule.forRoot(),
],
})
export class AppModule {}
And here is how we can use date picker and date range picker.
<div class="row">
<div class="col-xs-12 col-12 col-md-4 form-group mb-3">
<input
type="text"
placeholder="Datepicker"
class="form-control"
bsDatepicker
(bsValueChange)="onValueChange($event)"
/>
</div>
<div class="col-xs-12 col-12 col-md-4 form-group mb-3">
<input
type="text"
placeholder="Daterangepicker"
class="form-control"
bsDaterangepicker
(bsValueChange)="onValueChange($event)"
/>
</div>
</div>
onValueChange(value: any): void {
console.log('onValueChange', value);
}
As you can see these are just 2 directives which create date pickers from the input. We just need to provide a callback bsValueChange
where datepicker will provide a selection.
As you can see in browser we get date pickers out of box without any complexity.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done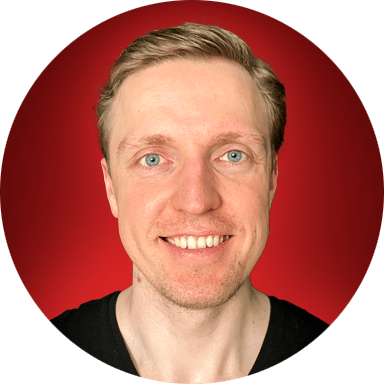