React Debugging in Vscode - No Browser Needed
In this post, I want to show you how to debug React in VSCode.
Here, I have already prepared a project for us to debug. It's a list of issues where we can select different issues, and at the top, we can see the number of selected issues.
Our project is running locally on localhost:5173
, which is important to remember for further debugging.
Typically, we use Google Chrome for debugging.
We can simply go to the sources, place a debugger inside, reload the page, and debug properties at that specific line with the breakpoint.
This is a totally valid approach, but you might want to do the same in VSCode to stay closer to your code and avoid changing your context.
Configuration
What are the pros and cons of this approach? Working inside your editor is more comfortable since you don't need to jump to the browser and back every time. How can we do that?
By clicking Cmd + Shift + D
, we can access the debug window on the left. Before running it, we must create a launch.json
file. You can see a link to create it at the top of the debugging window.
After clicking the link, we must select our debugger, which is Web App (Chrome)
. Here is how our file looks:
{
"version": "0.2.0",
"configurations": [
{
"type": "chrome",
"request": "launch",
"name": "Launch Chrome against localhost",
"url": "http://localhost:8080",
"webRoot": "${workspaceFolder}"
}
]
}
This file resides in the .vscode
folder. The type
is set to Chrome, and we must update the url
to the correct one. In our case, it's http://localhost:5173
. This will attach the debugger to our running project.
With our configuration in place, we can click Cmd + Shift + D
to activate debugging mode again.
It will open a separate Chrome window without our project. This window is bound to our VSCode.
At the top, we have buttons to work with the debugger.
Working with Debugger
How do we typically debug our application? In the file that we want to debug, we can place a debugger on the left side to add a breakpoint.
A breakpoint is a stop in the application. When we start the application, it will halt at this specific line, allowing us to debug this location.
On the left side, we see the debug window instead of our file tree. Additionally, you can see that our line is highlighted, indicating that we are stopped at this line.
What can we do here? On the left, we see our variables which we can inspect. Mostly, you want to look in the Local
variables section. Not only can we check the variables in the sidebar, but we can also hover over them in the code to see their values. We can open any item in the list to understand the problem in the specific record.
Furthermore, you can see all your imports on the left in the Module
section, as well as Global
properties in the respective section.
In 90% of cases, you are only interested in local variables.
Now, let's say that we don't know what's inside the issueEntries
and totalSelected
variables, and we want to check that.
We can place another breakpoint and click on the Continue
button in the development menu at the top of VSCode. This will execute all the code until our next breakpoint.
Here, we get access to the issueEntries
variable, which we can check in the sidebar. Additionally, we see the value of totalSelected
. This is how we can always know what values are there.
Conditional Breakpoints
Now, I want to stop debugging, so I'm clicking the Stop
button at the top of the debugging menu. Then, I right-click the breakpoint and select "Remove breakpoint" to remove it. Alternatively, we can right-click at the same place and select Add conditional breakpoint
.
Now, we can write some conditions with variables. For example, totalSelected === 0
. When you hover over the breakpoint, you can see that it's a conditional breakpoint. If we launch our debugging again and click Play
, we will come to our breakpoint because the totalSelected
variable is zero.
This is helpful when you have an array of items and you have a problem with one specific item.
How to use watch
Another important feature of debugging is how we can use watches. We can not only put a breakpoint but additionally can add an expression that we want to watch.
As you can see, we have access not only to the local properties but also to a watched expression, so we can know the value. Therefore, if you want to check some logic in a breakpoint, a watch expression is the way to go.
Callstack
Another thing you need to know about is the call stack. While it may not always be helpful, especially within frameworks, it can be useful for understanding your call flow in plain JavaScript.
The call stack is a list of files in a sequence of how they are called or imported into one another.
Here, we can click on each file and see what function is being called from it. Additionally, you can see breakpoints under the stack trace section where you can enable breakpoints related to specific files.
Buttons for debugging
When we start debugging, there is a debugging menu at the top of the file. Let's learn about all the buttons here. You already know play
- it continues executing our JavaScript. We also used Restart
to reload an application and Stop
to quit debugging.
But what about the other 3 buttons? Let's start with Step Over
. It jumps to the next line. It is important to remember that it doesn't jump inside functions, only across flat lines.
We also have Step Into
and Step Out
, which allow us to jump inside and outside functions to check values there.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
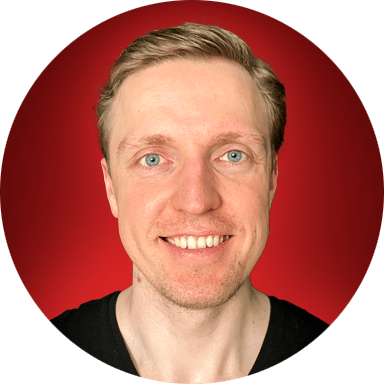