React State Management - All Ways and Solutions
In this post you will learn all popular solutions for state management in React.
Just from the start I want to say that there is no silver bullet. Every single solution here has it's use case.
useState
The first and the most popular solution is useState
hook. This is a possibility to create a local state inside a functional component with the usage of hooks.
We use useState
every single time when we need to create a local state.
This is totally fine but at some point you want to share this state between components. We don't have a possibility to share this local state. We can only pass it to our parent or our child.
We can't make useState global out of the box
This is why at some point it becomes really difficult to pass data through all levels of components.
React.Context
At this point you will typically check React.Context
. This is a possibility to make something accessible to the whole tree of our components. So you can just put your useState
or useReducer
inside global context and reuse it in any component without need to provide it inside props.
But here is a really important point. React.Context
is a low level tool. You implement yourself everything that you need. If you just added there a huge object and subscribed to it in all your components then all your components are rerendered at once. This is typically known as a render problem in React.Context
.
Jotai
Which brings us to the library which is the alternative to useState
but globally. This library is called Jotai.
It works in exactly the same way like useState
but you can use it across all your components and this is just a global client state inside your React project. Additionally inside this library they handled quite well problems with lots of renderings. You also don't need to wrap you code with providers every single time when you want global state like with React.Context
.
Jotai does just a client state like your theme or client filters.
Jotai doen't work with API or handle data from it in a comfortable way. It is not intended for that purpose.
React query
But working with API is tedious. Every single time we must write useEffect
, create a state and put sync API data with this state. Additionally we typically create error and loading states. If you want caching and invalidation of your data it is even more work.
This is why the most popular library to solve this case is React Query.
It synchronizes API data with local state inside your components.
Here in a single line with React Query we fetch data from the API and synchronize them to our local state with caching and invalidation out of the box.
But it is really important to distinguish between Jotai and React Query. Jotai is for client state management and React Query is for server state management. These 2 libraries are really a nice combo to implement big features inside your application by writing small amount of code.
Redux
The next thing here is something big, scalable but tedious to implement. I'm talking here about Redux. And not just Redux but the family of libraries where you have state management outside of the React which is working on top of the React.
Redux with Redux Toolkit is the most popular solution to implement big and scalable applications
Here we are writing lots of boilerplate code but it helps us to write all code in exactly the same way. In order to use Redux on a good level you must master lots of concepts like actions, action types, reducers, async actions, redux devtools and much much more but it is totally worth it.
Zustand
Additionally we have some alternatives to Redux which are kind of similar. The first alternative that people really like is called Zustand.
This is just an easier state management than Redux but it works in exactly the same way. You have some global state like in Redux, you also have functions which are similar to actions and when you make changes to your state it is similar to reducers in Redux.
As you can see on the image we can create some state and functions which will change this state. Then in our component we can access these properties in a hooks way.
Some people really like Zustand over Redux because you are writing less code and it is easier to learn it.
Mobx
The last alternative to Redux in the list is Mobx. It is the most different approach from the Redux because they are using there classes to make your application reactive.
But the main idea is the same. You have some state inside these classes, you can observe your state and you can make some mutations which change the state.
What should you learn?
The question now is for sure "But what should I learn?". First of all you must master React with usage of useState
hook. After that you for sure must try React.Context
to understand how it works. If you intend to apply to React job I highly recommend you to look on Redux and Redux Toolkit because this is the most popular solution which is used in lots of companies.
If you want to try something to write much less code I highly recommend you to look on Jotai + React Query.
But if you don't like Redux and looking for something similar or easier I recommend you to look on Zustand.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
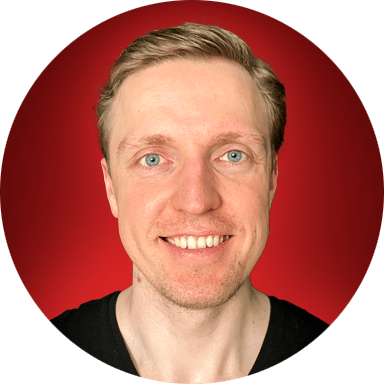