Responsive Registration Form in HTML and CSS
In this post we will build a responsive registration form just with plain HTML and CSS without any additional libraries.
Prepared project
Here I already prepared several files for us.
<!DOCTYPE html>
<html>
<head>
<title>Monsterlessons Academy</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="main.css" />
</head>
<body>
</body>
</html>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: inherit;
}
body {
display: flex;
align-items: center;
justify-content: center;
background: #037ef3;
padding: 50px 0;
}
This is just a basic html page with no specific CSS except of background and some resets.
Rendering markup
First of all we must start with preparing our markup. Here we will write our markup using BEM. If you don't know what is BEM it is a specific methodology how we can write CSS out of the box without any additional libraries.
It is called BEM because it is Block, Element and Modifier. You will see how we use it in a second.
<div class="form-container">
<div class="form-container__details">
<div class="form-container__title">Registration</div>
<div class="form-container__subtitle">
Welcome to Monsterlessons Academy
</div>
</div>
</div>
Here we created a block form-container
. We prefixed all child element with 2 underscored. These are elements of our block.
When we write code in such way we avoid problem with global styles as for us only blocks are global now.
Styling form-container
Let's add styles for our form-container.
.form-container {
width: 100%;
background: #fff;
padding: 30px;
max-width: 450px;
border-radius: 10px;
box-shadow: rgba(3, 3, 3, 0.1) 10px 0px 50px;
}
.form-container__details {
margin-bottom: 40px;
text-align: center;
}
.form-container__title {
font-size: 38px;
color: #555;
margin-bottom: 7px;
}
.form-container__subtitle {
font-size: 18px;
color: #999;
}
As you can see in browser our form container is correctly styled.
Form block
Now let's add our form block. And we want to create new block form
which is fully reusable and is not related to form-container
block.
<form class="form">
<div class="form__field">
<div class="form__label">Name</div>
<input class="form__input" />
</div>
<div class="form__field">
<div class="form__label">Email</div>
<input class="form__input" />
</div>
<div class="form__field">
<div class="form__label">Password</div>
<input class="form__input" type="password" />
</div>
<button class="form__submit">Register</button>
</form>
So here is the same approach. We create form__field
which has a label and an input inside. And all our child elements are prefixed with form
block.
And the last part is the bottom of our form. From my perspective it doesn't have anything to do with form
and it belongs to form-container
.
<div class="form-container__line-divider"></div>
<div class="form-container__links">
<a href="#" class="form-container__link">Login</a>
<a href="#" class="form-container__link">Forgot password</a>
</div>
Here we have our divider and links to different pages.
Styling form block
Now let's cover our form with styles.
.form__field {
margin-bottom: 30px;
}
.form__label {
margin-bottom: 10px;
font-size: 15px;
font-weight: 600;
color: #777;
}
.form__input {
width: 100%;
padding: 25px 15px;
border: 0;
background: #f0f0f0;
border-radius: 5px;
font-size: 18px;
color: #555;
font-weight: 600;
}
.form__submit {
background: #037ef3;
color: #fff;
font-weight: 600;
width: 100%;
border-radius: 5px;
cursor: pointer;
padding: 25px 15px;
border: 0;
transition: all 0.8s;
font-size: 18px;
}
.form__submit:hover {
background: #0271da;
}
We just styled our form-field
and it's child element. Now we can reuse a block form-field
with labels and inputs inside for any forms of our project.
The next step is to style bottom part of form container.
.form-container__line-divider {
background: #037ef3;
width: 30px;
height: 3px;
border-radius: 50px;
margin: 30px auto;
}
.form-container__links {
display: flex;
justify-content: space-between;
}
.form-container__link {
font-size: 15px;
font-weight: 600;
color: #777;
}
Now our form looks almost finished.
Responsive form
But we can add a few touches. If we make the page really narrow then our form doesn't have enough paddings to be good readable. Let's apply some media queries to fix it.
@media (max-width: 600px) {
.form-container {
padding: 30px 25px;
width: 90%;
}
.form__input,
.form__submit {
padding: 18px 15px;
}
}
Now our form is fully responsive.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done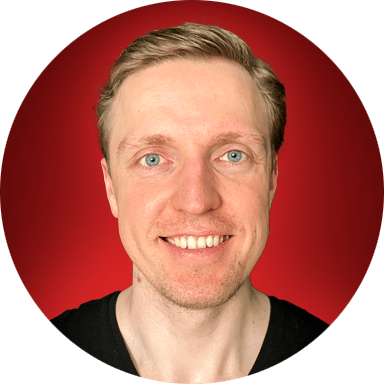