Super Clean Visual Studio Code Setup - Minimalistic Look
In this post, I want to show you how to configure the VSCode editor in a truly minimalistic way.
As you can see, I have a file tree with no tabs, no additional symbols, highlighting, or unnecessary distractions.
Many people simply install VSCode without configuring anything. At most, they install several plugins and leave it at that. However, by default, VSCode is quite bloated with features. While many of these features are useful, they push it more toward a fully-fledged IDE.
If you want to focus specifically on code, you might want to disable a lot of features to make it look cleaner and easier to read. This is why I prefer a minimalistic look for my editor while working. How can you achieve such a result?
Plugins
First of all, let's look at the extensions that I have installed. I have just five plugins, which are enough for me.
The first is "Angular Language Server." Since I use Angular quite a lot, this is a nice package that brings LSP support to VSCode.
The second is "Custom CSS and JS Loader." If you want some custom configurations that are not covered by VSCode's settings, you need this plugin because you can easily change elements by updating their CSS classes.
Next is "ESLint." Obviously, we want to see warnings from ESLint regarding problems with our code.
The fourth one is "Gruvbox Theme." This is the theme you can see on the screen, and it's what I use every day.
Another essential extension is "Prettier," which formats the code every time I save a file. This package is mandatory because it allows me to focus on the code instead of spending time on indentation.
The last one is "Subtle Match Brackets."
This extension provides an underline on the brackets that you can see in the editor. It's much more comfortable to view brackets this way rather than with the default VSCode brackets, as it looks more minimalistic.
Backup
Now we need to talk about backing up and reusing our settings on other machines. Imagine that you've configured your VSCode perfectly, but then you get a new computer and need to set it up again. You've already forgotten half of your settings, and it’s difficult to restore them. This is not an ideal situation, but fortunately, there are different solutions to this problem.
Inside Code -> Preferences -> Settings, there is a button labeled "Backup and Sync Settings." You can click here and select what you want to sync. Then, you create an account, sign in, and your settings will be backed up for you. This solution is great, especially if you are a beginner.
Another solution is to back up specific files and write your own scripts to restore them as part of your workflow. A typical example of such a workflow would be using dotfiles.
Dotfiles are a collection of files that provide configuration settings for various apps and tools. By copying them to a new machine, you can set up your environment in seconds.
For example, you can get a list of installed extensions like this
ls ˜/.vscode/extensions
This will show you a list of installed extensions.
cat ˜/Library/Application\ Support/Code/User/settings.json
And if you are looking for settings files, they are located here.
By backing up this file, you can always restore your settings on a new machine.
Starting From Scratch
What I did here was remove all configurations of VSCode and started from scratch.
Our goal now is to configure the same settings and show you which options I'm using and why.
The first step is to install all necessary plugins. Let's install all packages that I previously mentioned: "Prettier", "ESLint", "Gruvbox Theme" (choose "Dark Medium" for configuration), "Subtle Match Brackets", and "Angular Language Service".
It is much easier to write all configurations in a single file than to look for different options in the settings. Let's open our settings file.
code ˜/Library/Application\ Support/Code/User/settings.json
This file is empty except for the selected Gruvbox theme.
{
...
"window.zoomLevel": 3
}
The first option we want to change is zoomLevel
, as it makes the whole editor bigger and more readable.
{
...
"editor.formatOnSave": true,
"[html]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typescript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[css]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[json]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[javascript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[javascriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
}
}
The next option is formatOnSave
, so Prettier applies its format. Additionally, we set a list of extensions where Prettier must be applied.
{
...
"breadcrumbs.enabled": false,
"workbench.statusBar.visible": false,
"window.commandCenter": false,
"editor.minimap.enabled": false
}
We also want to remove breadcrumbs, the status bar, the minimap, and the command center to avoid clutter.
{
...
"editor.renderWhitespace": "none"
}
I don't want to see any white spaces in the code, so let's hide them.
{
...
"editor.fontFamily": "Monaco, Menlo, 'Courier New', monospace"
}
And I really prefer the default MacOS font, which is Monaco.
{
...
"workbench.activityBar.location": "hidden"
}
I also don't want to see the activity bar on the left. You might ask, "But how can I open the items that I clicked in the sidebar?" You just need to learn some hotkeys, like "Command + Shift + D" to open a debugger or "Command + Shift + E" to open an explorer.
{
...
"editor.tabSize": 2,
"editor.wordWrap": "on"
}
We need 2 spaces indentation, and wrapping works for comfortable work.
{
...
"editor.scrollbar.horizontal": "hidden",
"editor.scrollbar.vertical": "hidden",
"editor.hideCursorInOverviewRuler": true
}
I want to hide all scrollbars (don't worry, you can still scroll), and the lines that show changes in the vertical scrollbar.
{
...
"workbench.editor.tabActionCloseVisibility": false,
"workbench.editor.showTabs": "single"
}
Having lots of tabs is confusing and doesn't really help. I highly recommend sticking to a single tab and using Ctrl + P
to switch between recently opened files.
{
...
"explorer.decorations.colors": false,
"explorer.compactFolders": false
}
For the explorer, I prefer to see less highlighting and view all folders instead of the compact version.
{
...
"git.decorations.enabled": false,
"scm.diffDecorations": "none",
"scm.diffDecorationsGutterAction": "none",
"scm.diffDecorationsGutterPattern": {
"added": false,
"modified": false
},
"editor.glyphMargin": false
}
As I use console Git instead of Git in VSCode, I want to remove all Git help and highlighting from it.
{
...
"editor.bracketPairColorization.enabled": false,
"editor.guides.highlightActiveBracketPair": false,
"editor.guides.bracketPairsHorizontal": false,
"editor.matchBrackets": "never",
"editor.renderLineHighlight": "none",
"editor.showFoldingControls": "never",
"editor.folding": false,
"window.title": " ",
"editor.cursorBlinking": "solid",
"editor.cursorSmoothCaretAnimation": "on",
"editor.occurrencesHighlight": "off"
}
Here, we disabled default bracket highlighting and colorization, as well as highlighting the current line. We disabled code folding, removed the window title, cursor blinking, and added smooth animation to cursor movement.
Here is our end result. If you just want to apply the config without retyping it yourself, you can copy it from the source code at the end of the post.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done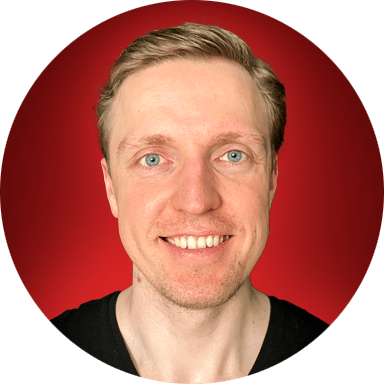