Things That I Want to See in JS
In this post I want to talk about things that I am really missing in JavaScript and I hope that people will implement them in future versions of it.
Static typing
And the first thing that I want to see in JavaScript is static typing. And actually people are using nowadays TypeScript just to bring static typing to JavaScript. But actually it is not a native way to create an application, because we simply write TypeScript code and when we transpile it to JavaScript.
const getName = (firstName: string, lastName:string): string => {
return firstName + ' ' + lastName
}
Which actually means we don't have TypeScript in production we still have JavaScript with all it's problems.
And actually if you are not familiar with TypeScript at all I highly recommend you to look on it. Because this is the future of JavaScript and more and more companies are using it every day.
So why do we need static type checking inside JavaScript at all? The main problem is that applications nowadays are much more complex, than they were before. We have all business logic and state management inside JavaScript. We also have single page applications, which doesn't make it easier. And it is much more difficult to develop an application just with plain JavaScript without any validation or data types.
And the main problem if you will compare TypeScript with for example C# is that we are losing all TypeScript typing when we are building production version of JavaScript as only JavaScript can be executed in browser. Which actually means all our data types are completely erased. And we have just plain JavaScript.
It is not like this in other typed languages, because for example inside Java or C# we have data types inside build version also.
Which actually means we can use data types in production.
In our case in JavaScript it is not possible. You can't use some data type and check the type of the variable and think that it will work later inside production. Because actually you will have there JavaScript and all of a data types are stripped off. And this is not convenient. This is why it would be really nice if we will have something like TypeScript by default with JavaScript.
// This won't work
if (foo is FooInterface) {
// do something
}
Transpiling
The next thing that I really hate is transpiling JavaScript to older versions. So I am talking here about using Babel or other transpilation tools which brings newer features inside JavaScript so we can use it already today across all browsers.
And this is all nice but transpilation really takes a lot of time. And the main problem here, that we are getting newer features inside JavaScript every single day. And all these browsers can't really support all these features out of the box. This is why we need transpilation of our code, so this code that we are writing with newer features will be transpiled in the browsers, for browsers where it is not yet supported.
But there are two main things that I hate about it. First of all it is impossible to debug. You have something like Webpack plus Babel to enable some new features and you don't have a clue how it is all transpiled and how you can debug it. Then you have some strange error with regenerator run time
for example or another tool which is used inside and you can spend hours just in debugging these issues.
Because the process of transpiling our code is extremely complicated. And output that you will see is also quite huge.
This is why what I really want to see is some other approach to work with JavaScript like in another languages. Obviously I want to use newer features of JavaScript, but I don't want to use transpilation until the end of my life.
Not enough functions
The next thing that really bothers me is the lack of good functions inside JavaScript itself. For example we can talk about working with URL or parsing query params. And here is the most popular package to parse query strings. It is called query-string
and actually people are using it in every single project. And you can see here the weekly downloads.
What this library does? It parses your query strings from location or it stringifies it. And this is it. And actually I expect this functionality to be implemented inside JavaScript itself. This is exactly what we need in every single project. It is not something that you will make only once in your life. So I really want to see a bunch of functions to do whatever I want with URL. For example read different parts like maybe location, hash, path name whatever you want or add params, remove params, generate URLs with params and whatever possible.
Exactly the same I can say about working with dates. Working with dates in JavaScript is extremely lacking and not comfortable at all. This is why a lot of people are using different libraries. For example the most popular ones are Luxon and Date-fns.
As you can see here for example we can write DateTime.now
. We are getting our local time, then we can set zone, so time zones are already there. Then we can write for example minus 1 week end of day. And we are getting out nice date in the readable format.
Exactly the same you can get if you are using other library date-fns. Here you can also generate dates, calculate intervals and work with time zones. But all this stuff doesn't exist out of the box inside JavaScript and there is no comfortable way to work with dates at all. And this is also something that I consider should be implemented on the level of JavaScript itself.
Data transformations
Another thing that bothers me a lot is working with data transformations inside JavaScript. We simply don't have enough methods to work efficiently and transform our data. For example let's look on something simple. We have sort function inside JavaScript and actually it is all nice, but here the usage.
const items = [
{name: 'Edward', value: 21},
{name: 'Sharpe', value: 37},
{name: 'And', value: 45},
{name: 'The', value: -12},
{name: 'Magnetic', value: 13},
{name: 'Zeros', value: 37},
]
items.sort((a, b) => a.value - b.value)
We are writing something like items.sort, where we are getting a and b. And here we are writing some magic with deduct. If you know how sorting is working then it is clear. You are getting something less than zero or bigger than zero, but it is not comfortable to read and use.
This is simply low level approach to implement sorting.
And yes you can implement whatever you want with this sorting. But typically people want to write their code easier. For example we can look on lodash library. This is one of two most popular libraries to do data transformations. And here we have a function, which is called orderBy. And here you can directly see the difference.
const items = [
{name: 'Edward', value: 21},
{name: 'Sharpe', value: 37},
{name: 'And', value: 45},
{name: 'The', value: -12},
{name: 'Magnetic', value: 13},
{name: 'Zeros', value: 37},
]
_.orderBy(items, ['value'], ['asc']);
We don’t have just a low level function. We have a orderBy function. And this is what you typically want, you have an array of objects and you want to sort them by some criteria. For example here we are writing orderBy and we simply set here as a second argument array of our keys. And this is exactly field by which we want to sort. As a third parameter we provide ascending or descending for each field accordingly. This is exactly super human readable, you don't really need to think a lot to understand this line.
But actually here when we are talking about native sort it is nice, but it is super low level. And the same I can say about hundreds of other functions inside libraries for data transformations. For example here inside Lodash we have lots of different functions. And the list is enormous. And actually I want to see at least 90% of such functions inside JavaScript. Because these things people use every single day. Things like capitalising or you want to compact your array and remove all nulls and underfined or maybe you want to find an intersection between two arrays.
You see these libraries for data transformation in every single project for a reason, because we are lack in the amount of good functions inside playing JavaScript.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
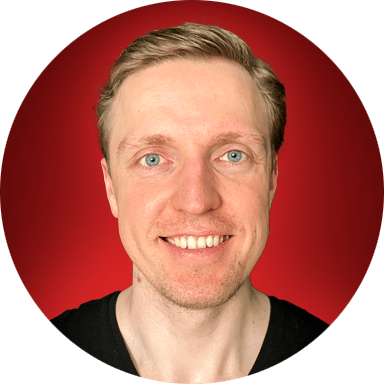