Typescript DTS File - What Is It For?
In this post you will learn why do you need d.ts
files inside Typescript and how to use it correctly.
So we typically just write some project with Typescript and here I already prepared a single file with some Typescript code.
function greet(person: string): string;
function greet(person: string[]): string[];
function greet(person: unknown): unknown {
if (typeof person === 'string') {
return `Hello, ${person}`
} else if (Array.isArray(person)) {
return person.map(name => `Hello, ${name}`)
}
throw new Error('Unable to greet')
}
console.log(greet(['Jack', 'John']))
It doesn't even matter if you fully understand this code. It is not the point of this post.
At some point we want to build our Typescript and transpile it to Javascript so it can be executed inside browser.
{
"devDependencies": {
"typescript": "^5.0.2"
}
}
So inside my package.json
I have a Typescript dependency so we can use it inside console.
./node_modules/typescript/bin/tsc
This is the Typescript command line tool which allows us to transpile code from the console.
./node_modules/typescript/bin/tsc src/main.ts --outfile build.js --declaration
This command transpiles our file src/main.ts
to build.js
and additionally created a declaration file. This is exactly this d.ts
file that we are talking about. It is being created only if we set a declaration
attribute.
Now let's check the output.
function greet(person) {
if (typeof person === 'string') {
return "Hello, ".concat(person)
} else if (Array.isArray(person)) {
return person.map(name => "Hello, ".concat(name))
}
throw new Error('Unable to greet')
}
console.log(greet(['Jack', 'John']))
As you can see our file was successfully transpiled to Javascript. This is plain Javascript and we can execute it in browser. We don't have any types inside the build file.
But additionally with this file we got a file with declarations
// build.d.ts
declare function greet(person: string): string
declare function greet(person: string[]): string[]
This is a Typescript file where we have just declarations from our Typescript code. It doesn't contain any functions or variables. Just types.
But why?
Now the question is why do we need this file at all? We have our Typescript, we successfully transpiled it to Javascript and we can execute it. This is totally file.
But just imagine that these file was a library. Our library was build with Typescript. But what we get as an output is Javascript.
If we import this library inside Typescript project we won't get any types because it was transpiled to Javascript.
The main problem with that is that we lose all Typescript definitions.
This is exactly why we need .d.ts
files. They have all typing definitions which will be used at the moment when we import our library Javascript to our Typescript file. Then we can read all these typings just fine.
So do we need to generate them always? If we want to import it later in Typescript projects. If you just need Javascript to execute it in browser you don't need d.ts
files. So mostly we need declarations either in libraries or in some reusable code that was build for internal needs.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
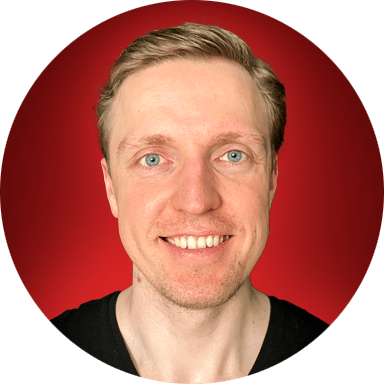