Typescript Function Overloading - Is It Good Enough?
In this post you will learn what is function overloading inside Typescript.
In a lot of different languages we have a feature which is called function overloading.
Function overloading is when you can create a single function but with different sets of arguments (different signatures)
We have something similar inside Typescript but it works a little bit strange. Inside Typescript we can have different signatures but only a single implementation.
It works like that only because Typescript is not a separate language. It is being transpiled to Javascript which means our code must be valid for both Typescript and Javascript.
Example
Let's have a look on a real example.
const greet = (person: string|string[]): string|string[] => {
if (typeof person === string) {
return `Hello, ${person}`;
} else if (Array.isArray(person)) {
return person.map(name => `Hello, ${name}`)
}
throw new Error('Unable to greet');
}
console.log(greet(['Jack', 'John']))
Here we have a greet
function which accept either string or array of string and returns different messages depending if it's an array or not.
As you can see when we call this function with an array we get an array of our messages back.
This implementation is totally fine and I always prefer such implementation in the code but you could also leverage function overloading to implement this function.
What does it mean? We either provide a string and get a string or provide an array and get an array back. This essentially means that we can have 2 different implementations of this function.
We could implement this in other languages with function overloading. But in Typescript it is different. We define several signatures and a single implementation.
function greet(person: string): string
function greet(person: string[]): string[]
This is how we can define several signatures in Typescript. Keep in mind that we can't use here arrow functions but we can only define them with function
keyword.
Now we must add just a single implementation of this function.
const greet = (person: unknown): unknown => {
if (typeof person === string) {
return `Hello, ${person}`;
} else if (Array.isArray(person)) {
return person.map(name => `Hello, ${name}`)
}
throw new Error('Unable to greet');
}
console.log(greet(['Jack', 'John']))
As you can see we simply changed argument to unknown
. The whole code stays the same. As we check for correct data types inside a function it works fine.
As you can see when I hover on the function call we see +1 overload
in the description.
Do you really need it?
Now the question is if you really need to use function overloading. No you don't. It is totally fine to write your functions without it as the implementation of function overloading in Typescript is anyway not the best one.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
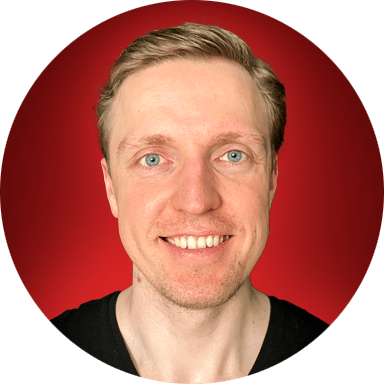