Vite React - Create React App alternative
In this post you will learn such tool which is called Vite and it can help you to make your development faster.
As you can see here is an official website of Vite and it says that it's a "Frontend tooling". It was built by a guy who created Vue which is Evan You.
What problem Vite solves?
Vite solves for us 2 different things. Vite creates for us a development server and it bundles all our frontend files for production.
Now you for sure thing that it doesn't make any sense to bring new tool if we have already Create-react-app, Webpack, Rollup or something similar.
But Vite works in much better way than these tools.
Vite is much much faster. So the main question now is how it can be faster? The main point is that Webpack any all other tools support older browsers.
As you can see inside CanIUse website es6 modules are not supported in older browsers this is why Webpack must do a lot of stuff bring support of this modules in older browsers.
This is one of the points why our building time is so slow especially when we have bigger projects.
Vite leverages usage of imports directly from the browser. Which actually means that it just does less.
Browser itself requests all modules and dependencies when we do import.
Vite gives files as it is to the browser from the backend when they are requested through HTTP.
Also Vite prebundles it's dependencies by using ESBuild tool which is written in Go. This is why it is much faster than Javascript build tools.
How it works
Now let's look how Vite works. To create new project we can use vite directly from npm.
npm create vite@latest .
Here I provided not a name of the project but . to initialize the project in current folder. If you don't want to do that you can specify the name of the folder.
npm create vite@latest vite-testing
As you can see here we can select a framework which we want to use. Vite can create for us a project with Vanilla JS, Vue, React, Svelte, Typescript and much more. As Vite was created by creator of Vue framework it works with it nicely. But it also works just fine with all these frameworks.
Vite is my go to tool to create React applications with and without Typescript.
But as you can see here we don't have Angular. It is not possible to generate Angular projects using Vite as codebase and complexity are just too high.
So here let's create a React application. And we can directly choose between Javascript and Typescript project.
This is awesome because Vite out of the box supports Typescript
Now we just need to install our dependencies and start a webserver. But here is an important point - the initial start of create-react-app project takes really a lot of time. For the small project it can take 10-15 seconds. For the big project it can be 1 minute.
My initial start of Vite project was 915ms which is extremely fast.
As you can see in browser the initial App component is rendered.
What Vite has inside?
Inside our project you can see src, public folder and index.html in the root. So it is not that difficult to jump from create-react-app to Vite.
Inside our index.html
we have not just a container for React project but we also load our first file as a es6 module.
Here you for sure want to ask how it works at all because we have a typescript file which can't be loaded in browser.
It works with Vite because it transpiles Typescript to Javascript on the fly.
Now let's look in network tab. Here we have lots of different chunks. For example we can see our App.tsx
and all it's dependencies will be automatically loaded in browser.
HMR
Now here is an interesting part. Do we have full page reload or hot mode reload? If we change something in our App.tsx
we can see DOM update directly in browser without page reload.
And the same goes with CSS. We can update our styles and it will be mirrored in our web page with HMR.
Type checking
If you are writing Typescript code there is one super important point. Vite uses inside EsBuild tool and it doesn't make any type checking in dev server.
You just get Typescript errors inside your editor because your editor runs Typescript server additionally to Vite.
interface User {
name: string;
}
const App = () => {
const user:User = {}
}
With this code we will directly see an error inside editor but we won't get any errors from Vite.
Vite doesn't do any type checking in dev time. This is why it is so fast.
But sure when we build our project to production we will get all Typescript errors as normal.
Vite & node_modules
The last question that we need to clarify is how does it work with node_modules. We all know that es6 modules in browser work just with relative path and don't work when required from node_modules.
Our browser doesn't know anything about node_modules and how they work.
Even import of useState
from React is not fine. This is exactly what Vite does for us. It checks all node_modules dependencies and resolves them correctly.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
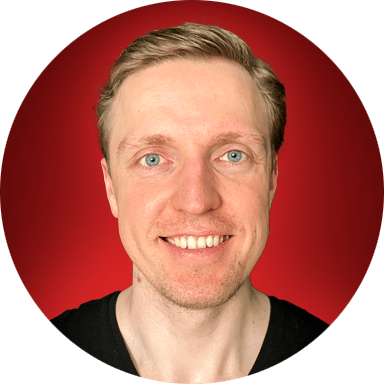