Work with Javascript Query Params effectively without Libraries
In this post I will show you how to work effectively with query parameters in Javascript without any additional libraries.
Previously I always used some library in order to work with query parameters. Like for example query-string
which allows you to parse url, convert it to Javascript object, stringify and much more. Inside Javascript previously we didn't have any comfortable methods to do that.
But now we have URL API which works just fine in all modern browsers.
So if you are not supporting older browsers like for example IE11 or before you are totally fine. And now I want to show you most popular cases that we need every day and how you can solve them.
Add params to url
The most common case that we need to solve it adding query parameters to the url.
const testUrl = "https://www.example.com/page";
const url = new URL(testUrl)
url.searchParams.append('foo', 'foo')
url.searchParams.append('bar', 'bar')
console.log(url.toString())
Here we have our testUrl
. We create an instance of URL
which allows us to change our url. We use searchParams.append
to add parameters and we set them as key and value.
https://www.example.com/page?foo=foo&bar=bar
As you can see we got our base url with appended query parameters. It is important to remember that url
is an instance and not just a string. In order to get a string to must always call toString
method.
Remove all query params
Another important questing it how to move all query parameters and get just a base url. Typically we split the url and get a first element.
const testUrl = "https://www.example.com/page?foo=foo";
console.log(testUrl.split('?')[0])
This code works but it is ugly and doesn't leverage URL
class.
const testUrl = "https://www.example.com/page?foo=foo";
const url = new URL(testUrl)
url.search = ''
console.log(url.toString())
This code might look a bit longer but it is easier to read and we directly see that we set the whole search to an empty string.
https://www.example.com/page
Remove single query param
But what should we do if we have several query params but we just want to remove one?
const testUrl = "https://www.example.com/page?foo=foo&bar=bar";
const url = new URL(testUrl)
url.searchParams.delete('foo')
console.log(url.toString())
With the help of searchParams.delete
we can remove just a single query parameter.
https://www.example.com/page?bar=bar
Parsing query params
Another important case is to parse all query parameters and convert them to Javascript object. In this case we can work with them efficiently.
const testUrl = "https://www.example.com/page?foo=foo&bar=bar";
const url = new URL(testUrl)
const params = Object.fromEntries(url.searchParams.entries())
console.log(params)
With the help of fromEntries
function we can convert query parameters to the object.
{foo: 'foo', bar: 'bar'}
Stringify parameters to url
The last case that we need is to stringify params back to the url after we did manipulations with them in Javascript.
const params = {foo: 'foo', bar: 'bar'}
const searchParams = new URLSearchParams(params)
const newUrl = `http://localhost:3000?${searchParams.toString()}`
Here we used URLSearchParams
to convert Javascript object to query parameters.
http://localhost:3000?foo=foo&bar=bar
As you can see we are getting correct stringified url.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
📚 Source code of what we've done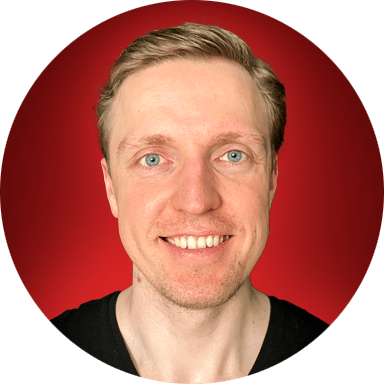