Working With Git in Visual Studio Code - Pushing to Github Visual Studio Code
In this post, I have prepared a Git + VSCode tutorial to help you use it efficiently within your favorite editor.
Changing Hotkey
As an initial project, I created a completely empty folder without Git, containing only a single main.js
file. To start working with Git, activate the "Source Control" tab on the left. You can do this by selecting View -> Source Control
. However, the hotkey for this is inconvenient: while Explorer uses Cmd + Shift + E
and Search uses Cmd + Shift + F
, Source Control is, for some reason, Ctrl + Shift + G
.
We can go to keybindings and change it to Cmd + Shift + G
. Now, to access "Source Control," we can simply click Cmd + Shift + G
.
Basic Workflow
The first thing we see on the left is an initial panel about Git.
Here, Git is not initialized yet. Let's click on the "Initialize Git" button.
It's the same as writing
git init
in the console.
What we see now on the left is a list of files that have changed. Here, we have just a single file, main.js
. If we click on it, we see our changes, where additions are green and deletions are red.
Additionally, at the top of the file, we see a marker labeled "Untracked." This means that this file is not yet tracked by Git.
When we hover over "Changes" in the left sidebar, you can see several buttons. You have "View Changes" and "Rollback."
Now, let's create a new file, todos.js
.
console.log('todos')
Let's jump back to the "Source Control" panel.
Now, we see two changed files.
Another important button under "Changes" is "Stage All Changes." This means adding all your changes to Git. Yes, we want this, so let's hit this button.
As you can see, these files have been moved to the "Staged" section. Now they are not only tracked by Git but also added to the index for our future commit. We can now enter a commit message and click "Commit."
Now we don't see any files, only a "Publish" button, which indicates that we have successfully created a commit. This is the state where none of our files are changed.
Status Bar
Another important thing to remember when working with Git inside VSCode is to enable the status bar in the settings. It allows you to work with Git in a more efficient way.
Now on the bottom, you can see Git information about the current branch. You can click on the branch name to open a menu with several options.
If you're implementing a new feature, what you typically want to do is click "Create New Branch" and provide a name for the branch there.
Now in the status bar, we can see that we are on another branch. When we click on the branch in the status bar, we can jump to another existing branch or create a new one.
More Changes
Let's make some more changes. I want to update our main.js
file.
// todos.js
const renderTodos = () => {
console.log('renderTodos')
}
// main.js
import {renderTodos} from './todos'
renderTodos()
Let's take a look at our Git status.
In the "Changes" section, we see modified files marked with the letter "M". On the right, we can see all our changes with additions and deletions. Let's add both files to the commit and create one more commit.
Now, the question is how we can see all our commits inside VSCode.
To view commits, you need to activate "Explorer" on the left and open "Timeline" at the bottom left. By default, it shows not only Git commits but also local file changes. To filter them out, you can disable local history and leave only Git history. You can also click on a commit to see what changes were made in that specific commit.
Pushing to Github
We can already see our commits, but how do we deploy them to GitHub? It's not sufficient to work with Git locally; we need to push our code to a remote repository. To do this, we must click the "Publish Branch" button that appears after committing. Afterward, we need to click "Allow" to authorize VSCode to access our repository.
In VSCode, we are asked whether we want to push to a private or public repository. I want to select private. In the bottom right corner, we will receive a message that our code is published, and we will be asked if we want to run "Git fetch" periodically. Yes, we do.
When we navigate to our GitHub, our code is pushed there along with all branches. If we make more changes and commit them, we will see a "Sync Changes" button, which will publish all changes to GitHub.
Advanced Usage
Now you know the basic workflow with Git. If you need more, there is access to a lot of Git commands in VSCode.
Additionally, in addition to all these commands, if you are curious about what Git does under the hood, you can always click on the "Show Output" command.
Now, let's say that we want to merge our changes from the feature branch to main
. We can switch to the "main" branch in the status bar, open the Command Palette, and type "Git merge" there.
And select the branch that we want to merge into "main". Let's hit Enter, and all our changes are now in the "main" branch.
Want to conquer your next JavaScript interview? Download my FREE PDF - Pass Your JS Interview with Confidence and start preparing for success today!
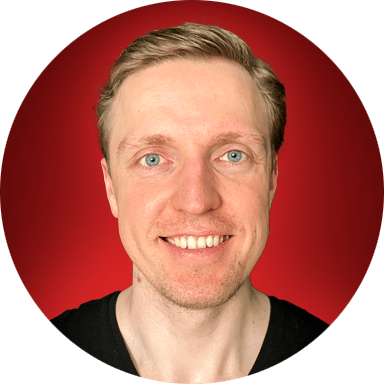